Creating a Telegram Bot
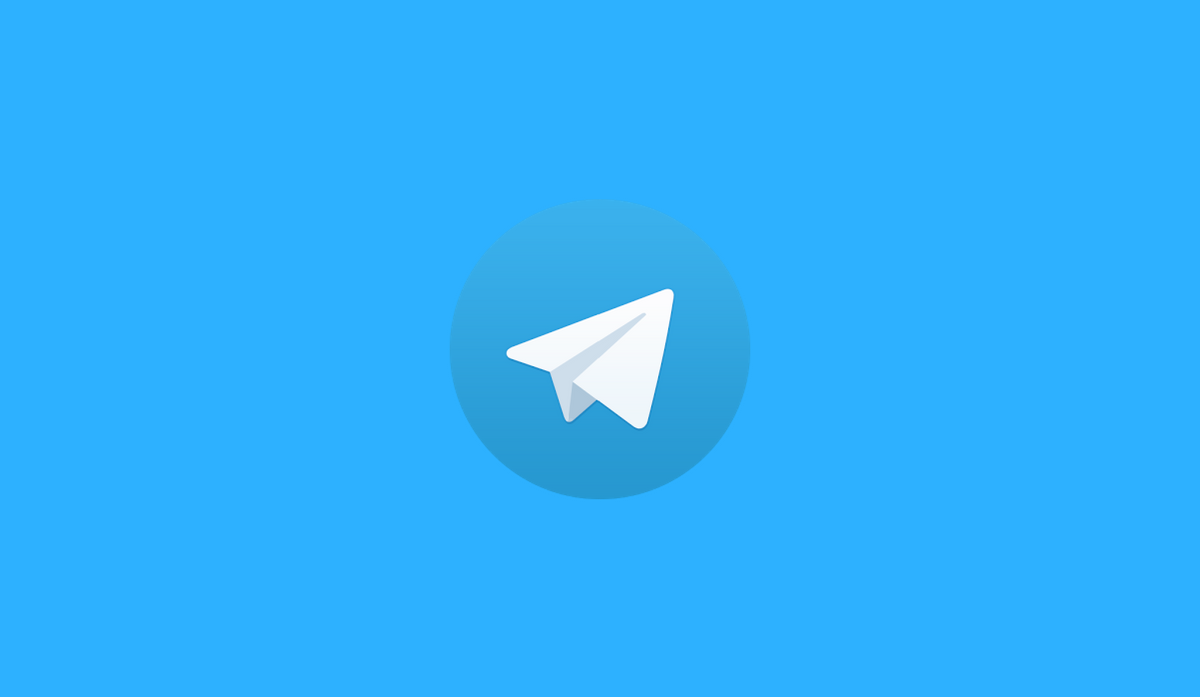
First, you must have or create a Telegram account. Second, you’ll need to create a Telegram Bot in order to get an Access Token. You can do so by talking to the BotFather and following a few simple steps.
Telegram Bots can receive messages or commands. The former is simply text that you send as if you were sending a message to another person, while the latter are prefixed with a
/
character.
To create a new bot, send the /newbot command to BotFatheras a chat (exactly as if you were talking to another person on Telegram).
You should get a reply instantly that asks you to choose a name for your Bot. You have to send then the name you want for the bot, which can be anyone, for instance, “RTelegramBot”.
BotFather will now ask you to pick a username for your Bot. This username has to end in “bot”, and be globally unique. After sending your chosen username to BotFather, it will send you a “Congratulations” message, which will include a token. The token should look something like this:
123456:ABC-DEF1234ghIkl-zyx57W2v1u123ew11
For the rest of this tutorial, we’ll indicate where you need to put your token by using.
TOKEN
Take note of it, as you’ll need it in the code that you are about to write.Storing and retrieving your Token
Following Hadley’s API guidelines it’s unsafe to type the
TOKEN
just in the R script. It’s better to use environment variables set in .Renviron file.
So let’s say you have named your bot RTelegramBot; you can open the . Renviron file with the R commands:
file.edit(path.expand(file.path("~", ".Renviron")))
And put the following line with your
TOKEN
in your.R environ:R_TELEGRAM_BOT_RTelegramBot=TOKEN
Finally, restart R in order to have working environment variables.
Creating a Bot instance
To start playing with your new Bot, you should install the telegram.botpackage and create an instance of the Bot class.
# install.packages("telegram.bot") library(telegram.bot) bot <- Bot(token = bot_token("RTelegramBot"))
If you followed the suggested
R_TELEGRAM_BOT_
prefix convention seen in the previous section, you’ll be able to use the bot_token function, otherwise, you’ll have to get these variables from Sys.getenv.print(bot$getMe())
Bear in mind that Bots can’t initiate conversations with users. A user must either add them to a group or send them a message first. Thus, search your Bot username in any of the Telegram clients and start a conversation with it.
After you sent the first message, you can get updates from your bot with the command:
updates <- bot$getUpdates()
This will retrieve a list generated from the JSON response from the server. In order to send a response, you can do it so with the following commands:
chat_id <- updates[[1L]]$message$chat$id bot$sendMessage(chat_id = chat_id, text = "Hello!")
Develop a Telegram Chatbot with R
In order to build a bot that is continuously running and is able to respond to multiple input data formats, also known as “chatbot”, the telegram.botpackage features several R6 classes, but the two most important ones here are Updater and Dispatcher.
The Updater class continuously fetches new updates from Telegram and passes them on to its Dispatcher class. You can then register handlers of different types in it so to deliver the fetched updates to a callback functionthat you defined. Every handler is an instance of any sub-class of the Handlerclass.
Finally you can run the chatbot with the method start_polling, which runs a loop that has the following structure:
- Get updates through the getUpdates method using long polling.
- Dispatch each update to the appropriate process function.
- Send an answer if specified in those functions.
Below we explain how to build a bot with this structure.
Building your first R Chatbot in 3 steps
The best way to get familiar with these concepts is to put them in practice with an example.
1. Creating the Updater object
Let’s start by creating the Updater object:
updater <- Updater(token = bot_token("RTelegramBot"))
So far, so good, don’t you think?
2. The first functions
Now we are going to build two handlers for our chatbot. The first one will handle updates every time the Bot receives a Telegram message that contains the /start command. To accomplish that, you can use a CommandHandler (one of the provided Handler sub-classes) and register it in the updater’s dispatcher (which is done with the
+
operator):start <- function(bot, update) { bot$sendMessage(chat_id = update$message$chat_id, text = sprintf("Hello %s!", update$message$from$first_name)) } start_handler <- CommandHandler("start", start) updater <- updater + start_handler
As you can see, the handler’s callback functions require two arguments: bot, which represents the Telegram Bot associated to our updater; and update, representing an incoming update.
Moving forward, let’s define another handler that listens for regular messages. Use the MessageHandler, another Handler sub-class, to echo to all text messages:
echo <- function(bot, update) { bot$sendMessage(chat_id = update$message$chat_id, text = update$message$text) } echo_handler <- MessageHandler(echo, MessageFilters$text) updater <- updater + echo_handler
From now on, your bot should echo all non-command text messages it receives. This has been told with the MessageFilters object, which contains a number of functions that filter incoming messages for text, images, status updates and more. Any message that returns TRUE for at least one of the filters passed to MessageHandler will be accepted. You can also write your own filters if you want.
3. Starting the Chatbot
And that’s all you need. To start the bot, run:
updater$start_polling()
Give it a try! Start a chat with your bot and issue the /start command - if all went right, it will reply. Try also texting and see what it responds!
Code summary and conclusion
And that’s all for now! If you want to learn more about Telegram Bots with R, you can check out Telegram’s documentation Bots: An introduction for developers and Telegram Bot API to familiarize with the API.
The code used to build our chatbot would be, in short:
library(telegram.bot)
# start handler function start <- function(bot, update) { bot$sendMessage(chat_id = update$message$chat_id, text = sprintf("Hello %s!", update$message$from$first_name)) }
# echo handler function echo <- function(bot, update) { bot$sendMessage(chat_id = update$message$chat_id, text = update$message$text) }
# build the updater updater <- Updater(token = bot_token("RTelegramBot")) + CommandHandler("start", start) + MessageHandler(echo, MessageFilters$text)
# start polling updater$start_polling()
With this tutorial you may have a global overview of the Telegram Bot API and the first guidelines to develop your own chatbot with R.
I hope you enjoyed it!
Recommended Ebooks :
Hands-On Programming with R: Write Your Own Functions and Simulations
Machine Learning with R - Second Edition: Expert techniques for predictive modeling to solve all your data analysis problems
The Book of R: A First Course in Programming and Statistics
2 Comments
Excellent and very cool idea and the subject at the top of magnificence and I am happy to this post..Interesting post! Thanks for writing it. What's wrong with this kind of post exactly? It follows your previous guideline for post length as well as clarity..
ReplyDeleteChatbot Company in India
Chatbot Company in Chennai
Chatbot Development Company in Chennai
Chatbot in Chennai
Chatbot Development Company in India
Wonderful blog & good post.Its really helpful for me, Your blog is easily understandable and give complete information. Keep sharing new ideas and features.
ReplyDeleteChatbot Developers in Dubai
Facebook Chatbot in Dubai
AI Chatbot in Dubai
Artificial intelligence Company in Dubai